Getting Started with Git: Common Commands
If you’re new to Git or need a refresher on some of the most commonly used commands, this section will provide you with a quick overview. Git is a powerful version control system used by developers to track changes in their projects. Below are some fundamental Git commands to help you get started.
Houda Srhir
3/20/20247 min read
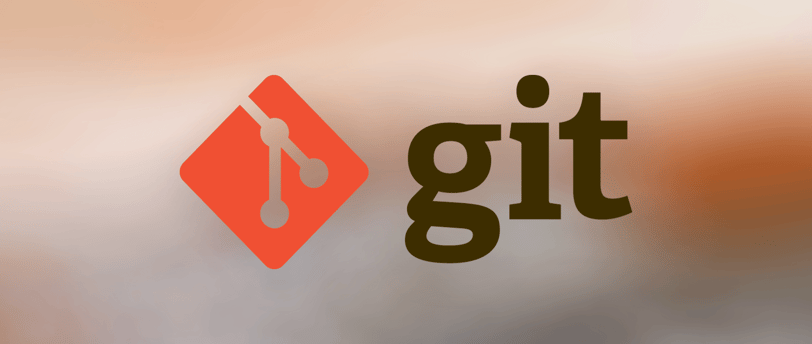
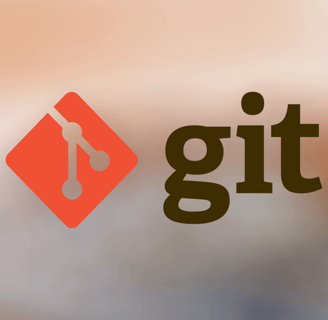
1. Initializing a Repository and Setting Up a Remote Connection
To start using Git in a project, you’ll first need to initialize a repository.
$ git init : This command initializes a new Git repository in the current directory, setting up all the necessary files and directories for version control.
If you’re working with a project that already exists on a remote repository and you want to connect your local repository to it, you can use git remote add to specify the remote URL.
$ git remote add <name> <url> : This command allows you to connect your local repository with a remote repository.
<name> is a label you provide for the remote repository. It’s often called “origin”, but you can choose any name you want.
<url> is the URL of the remote repository.
2. Cloning a Repository
If you want to work on an existing project hosted on a remote repository, you can clone it to your local machine using git clone
$ git clone <repository_url> : This command is used to create a copy of an existing Git repository.
<repository_url> is the URL of the remote repository you want to clone.
3. Staging and Committing Changes
Once you’ve made changes to your project files, you’ll need to stage and commit those changes to Git.
$ git add <file> : This command is used to add specific changes in a file to the staging area (also known as the index).
<file> is the name of the file you want to add to the staging area.
$ git add -A or $ git add — all : This command tells Git to stage all changes, including modifications, deletions, and new files, in the entire working directory and its subdirectories.
Once you’ve added all the changes you want to include in the next commit, you can proceed to commit them using git commit.
$ git commit -m “The commit message” : This command is used to record the changes that are staged in the staging area to the repository.
You can also use git commit -am “The commit message”command to stage all modified and deleted files in the working directory and commit them with the provided commit message in a single step.
4. Git Commands for Collaboration and History Tracking
Git provides several commands to view changes and the commit history of your repository. Here are a few useful ones:
$ git status : This command is used to display the current state of the working directory and the staging area in your local Git repository. When you run git status, Git will show you:
· Which files have been modified since the last commit.
Which new files have been created and are not yet tracked by Git.
* Which files have been staged for the next commit.
* Which branch you are currently on.
$ git diff : The git diff command is used to show changes between commits, the index, or the working directory. It’s a powerful tool for understanding what has changed in your repository.
some common use cases of git diff:
$ git diff — staged : This command shows the difference between the changes in the staging area (index) and the last commit.
$ git diff <commit1> <commit2> : This command shows the difference between two commits. You can replace <commit1> and <commit2> with the commit hashes, branch names, or tags you want to compare.
$ git diff <file> : This command shows the changes made to a specific file since the last commit. Replace <file> with the filename you want to inspect.
$ git diff <branch1>..<branch2> : This command shows the difference between two branches. It’s equivalent to $ git diff branch1 branch2.
$ git log : This command is used to display the commit history of a Git repository. When you run git log, Git will display a list of commits in reverse chronological order, starting from the most recent commit. Each commit is listed with its unique identifier (hash), author, date, and commit message.
You can customize the output of git log using various options, such as — oneline and — graph.
$ git log — graph : to show a text-based graphical representation of the commit history, and many others.
$ git log — oneline : to display each commit on a single line, — graph
$ git blame <file_path> : This command is used to display the revision and author of each line in a file, showing who last modified each line and in which commit.
5. Branching and Merging
Branching and merging are essential features of Git that allow you to work on multiple features or fixes simultaneously and integrate changes from different branches seamlessly.
Git provides commands to create, list, switch between, and delete branches:
$ git branch <branch_name> : This command create a new branch git branch.
$ git branch : This command List all branches in the repository
$ git checkout <branch_name> : This command switch to a different branch.
$ git checkout -b <new_branch_name> : This command create a new branch and switch to it in one step.
$ git branch -d <branch_name> : This command delete a branch (use -d to prevent deletion if changes are not merged).
Merging changes
Once you’ve made changes in a branch and want to incorporate them into another branch, you’ll need to merge them:
$ git merge <branch_name> : This command merge changes from another branch (<branch_name>) into the current branch.
Git will attempt to automatically merge the changes, but if there are any conflicts (i.e., changes that conflict with each other), you’ll need to resolve them manually.
After resolving any conflicts, you can complete the merge by committing the changes.
6. Remote Operations
Git allows you to interact with remote repositories, such as those hosted on platforms like GitHub, GitLab, or Bitbucket. You can push your local changes to a remote repository, fetch updates from a remote, and more.
To work with remote repositories, you’ll need to add them to your local Git configuration:
$ git remote add <remote_name> <repository_url> : Add a remote repository with a name.
$ git remote -v : List all remote repositories
$ git remote rename <old_name> <new_name> : Rename a remote repository
$ git remote remove <remote_name> : Remove a remote repository
Once you’ve added a remote, you can push your local commits to it or pull changes from it:
$ git push <remote_name> <branch_name> : This command push commits from the current branch to a remote repository.
$ git pull <remote_name> <branch_name> : This command pull changes from a remote repository and merge them into the current branch.
$ git fetch <remote_name> : This command fetch changes from a remote repository (does not merge them into the local branch).
7. Undoing Commits
There may be situations where you need to undo or modify previous commits in your Git history. Git offers several methods to accomplish these tasks, depending on your specific needs.
If you need to undo multiple previous commits, you can use git reset or git revert:
$ git revert <commit> : This command is used to create a new commit that undoes the changes made by a previous commit.
<commit> is the identifier (hash) of the commit you want to revert.
The git reset command is used to undo changes, move the HEAD to a different commit, or unstage changes, depending on how it’s used and which options are provided.
some common use cases of git reset:
$ git reset <commit> : This moves the HEAD to the specified commit, resetting the staging area and the working directory to match that commit.
$ git reset — soft <commit> : This resets the HEAD to the specified commit but keeps the changes staged. It doesn’t affect the working directory,
so the changes remain there for further modifications.
$ git reset — hard <commit> : This resets the HEAD to the specified commit, discarding all changes in the staging area and working directory.
It’s a destructive operation and should be used with caution, as it permanently removes changes.
$ git reset — mixed <commit> : This resets the HEAD to the specified commit, leaving changes in the working directory but unstaged. It effectively removes changes from the staging area.
For example, git reset — soft HEAD~1 will undo the last commit, git reset — soft HEAD~2 will undo the last two commits, and so on.
8. Managing Work in Progress
During development, you may encounter situations where you need to temporarily set aside changes without committing them.
git stash command is used to temporarily store changes that are not ready to be committed, allowing you to work on another task without committing incomplete changes to your repository.
how to use git stash:
$ git stash : This command stashes (saves) your local modifications, including both staged and unstaged changes, into a stack of stashes. After running this command, your working directory will be clean, as if you haven’t made any changes.
$ git stash list : This command shows a list of stashes you’ve created, along with a reference (stash@{n}) for each stash.
$ git stash apply : This command reapplies the most recent stash’s changes to your working directory. The changes are applied without removing the stash from the stash list.
$ git stash pop : This command applies the most recent stash’s changes to your working directory and then removes the stash from the stash list.
$ git stash apply stash@{n} : This command applies the changes from a specific stash referenced by its index (stash@{n}) to your working directory.
$ git stash drop stash@{n} : This command removes a specific stash from the stash list without applying its changes to your working directory.
9. Configuring Git Settings
Customizing Git settings is essential for tailoring your workflow to your preferences and requirements. Git provides the git config command to manage configuration settings at various levels, from global user settings to repository-specific configurations.
Common usage includes:
$ git config — global user.name “Your Name” : This sets your user name globally for all repositories on your system.
$ git config — global core.editor “textEditor” </code>: This sets your default text editor for commit messages and other Git operations. Replace “textEditor” with your preferred text editor.
$ git config — list : This displays the current Git configuration.
$ git config — global alias.co checkout : This creates a shortcut alias co for the checkout command.
In summary, Git is a powerful version control system that offers a wide range of commands and features to streamline the development process. By mastering essential Git commands such as initializing repositories, staging changes, branching, and managing configurations, developers can efficiently track changes, collaborate effectively, and maintain organized project histories. With Git’s flexibility and robust functionality, teams and individuals alike can navigate development challenges with confidence, ensuring the success of their projects.