Mastering Spring Data JPA: A Comprehensive Guide to Data Access in Spring Boot
This article serves as a guide to using Spring Data JPA within a Spring Boot application. It covers configuring JPA, setting up a connection to a database, defining entities and repositories, and implementing various queries. The guide also discusses advanced features like event listeners, custom repositories, auditing, and Spring Data REST for RESTful endpoint exposure. It provides code examples and a clear explanation of concepts to help developers of all levels effectively leverage Spring Data JPA in their Java web projects.
Houda Srhir
4/14/20243 min read
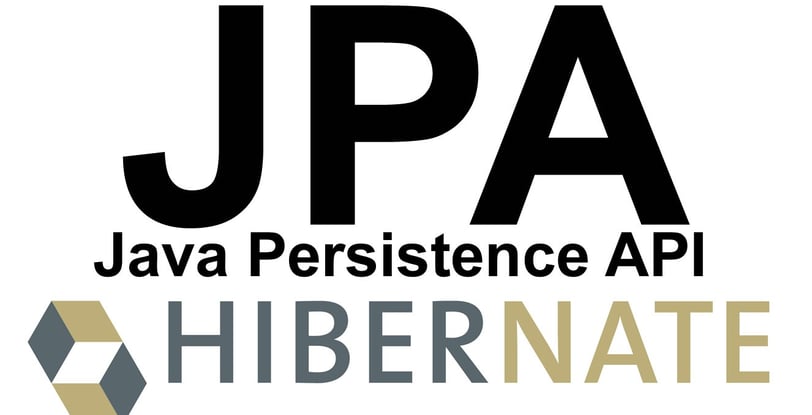
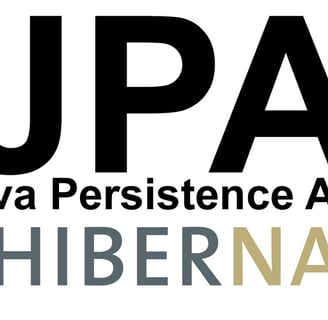
In the world of Java web development, the Spring Framework has long been a cornerstone, offering developers robust tools and frameworks for building enterprise-grade applications. Spring Data JPA, a part of the Spring Data project, simplifies data access in Spring applications by providing a unified and consistent programming model for interacting with various data sources.
Introduction to Spring Data JPA and Spring Boot:
Spring Data JPA, when used in conjunction with Spring Boot, provides a seamless and efficient way to interact with databases in Java applications. Key aspects of this integration include:
Annotation-driven configuration: Spring Data JPA relies on annotations like @Entity and @Repository for configuring entities and repositories, respectively.
Repository interfaces: You define repository interfaces extending the JpaRepository or its sub-interfaces, which come with built-in methods for basic CRUD operations.
Query methods: You can define custom query methods in repository interfaces using method name conventions or explicit JPQL, Criteria API, or even native SQL queries.
Pagination and sorting: Spring Data JPA offers built-in support for paginating and sorting query results, simplifying the implementation of paginated data access.
Integration with Spring Boot: Spring Boot's auto-configuration and starter dependencies streamline the setup and usage of Spring Data JPA.
1. Setting up a Spring Boot project with Spring Data JPA:
To set up a Spring Boot project with Spring Data JPA, follow these steps:
1.1. Add the spring-boot-starter-data-jpa and a database driver dependency (e.g., H2, MySQL, or PostgreSQL) to your pom.xml file.
1.2. Configure the database connection properties in the application.properties or application.yml file.
1.3. Enable JPA repositories in your Spring Boot application by adding the @EnableJpaRepositories annotation to your main configuration class, specifying the package where your repository interfaces reside.
2. Define Entity Classes:
Create Java classes representing the entities you want to persist in your database. Use JPA annotations to map class properties to database table columns.
3. Defining Repository Interfaces
Create repository interfaces for interacting with entities. Extend either CrudRepository, JpaRepository, or PagingAndSortingRepository, specifying the entity type and its primary key type. Add the @Repository annotation to make Spring aware of the repository.
4. Usage of Repository Methods:
Inject repository interfaces into services or controllers to interact with your application's data:
In controllers, you can use the service class to handle data operations and return responses to the client:
5. Fetching Strategies and Relationships
Spring Data JPA supports different fetching strategies to control when associated entities are loaded from the database:
1. Eager Fetching:
Associated entities are loaded immediately along with the owning entity. Use `@OneToMany(fetch = FetchType.EAGER)` or `@ManyToOne(fetch = FetchType.EAGER)` to specify eager fetching.
2. Lazy Fetching:
The associated entity is not fetched immediately. It is fetched only when a getter method is called. Use `@OneToMany(fetch = FetchType.LAZY)` or `@ManyToOne(fetch = FetchType.LAZY)` for lazy fetching.
For many-to-many relationships, you can use an `@ManyToMany` annotation with `@JoinTable` to define the relationship table.
By following these steps and understanding the concepts, you can effectively use Spring Data JPA within a Spring Boot application to manage your database interactions in a clean, organized, and efficient manner.
6. Advanced Features and Customization
1. Event listeners:
Spring Data JPA supports event listeners for handling events during the entity lifecycle, such as before or after an entity is saved, updated, or deleted. You can create a class that implements the `ApplicationListener` interface and specify the event class (e.g., `@Component` and `@EventListener`). For example:
2. Custom repositories:
If the built-in repository methods are not sufficient, you can create custom methods and query implementations. You can extend `JpaRepository` or `CrudRepository` and add your custom methods. For example:
3. Auditing:
Spring Data JPA can be integrated with Spring Data Auditing to track changes made to entities. Add the `@EnableJpaAuditing` annotation to your configuration class, and annotate entity fields you want to track with `@CreatedDate`, `@LastModifiedDate`, and `@CreatedBy`. For example:
4. Spring Data REST:
If you want to expose your repositories as RESTful endpoints, you can use Spring Data REST. Add the `spring-boot-starter-data-rest` dependency and configure your application. This will automatically expose your repositories through a simple REST API.
By exploring these advanced features and customizations, you can further tailor your Spring Data JPA integration to meet the specific needs of your application. As you become more familiar with the framework, you'll be able to leverage its power to build scalable and maintainable Java web applications.