Unlocking Java Excellence: Introduction to Aspect-Oriented Programming with Spring
This comprehensive guide introduces readers to the world of Aspect-Oriented Programming (AOP) with a focus on Spring AOP, a powerful paradigm for enhancing code organization and maintainability in Java applications. The article covers core concepts of AOP, including aspects, join points, advice types, and supported pointcut designators. It provides a step-by-step guide on implementing AOP in a Spring Boot project, along with practical examples illustrating its application. By leveraging Spring AOP effectively, developers can simplify development tasks, improve code quality, and enhance the overall functionality of Java applications.
Houda Srhir
3/23/20244 min read
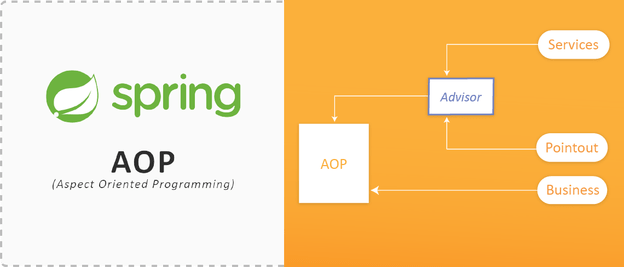
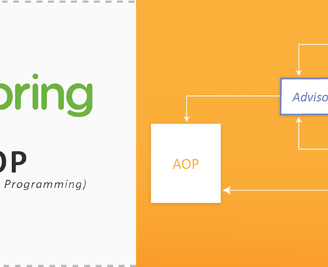
In the realm of software development, maintaining clean, modular, and scalable code is crucial. However, as applications become more complex, managing common concerns across multiple components becomes increasingly challenging.
Aspect-Oriented Programming (AOP) Core Concepts:
AOP is a paradigm used to encapsulate and modularize cross-cutting concerns like transaction management, logging, and security. It provides a systematic way to address these concerns, enhancing code organization and maintainability.
Aspect: An aspect encapsulates a cross-cutting concern, such as logging or security. These aspects act as reusable modules applied across different parts of an application.
Join point: This refers to a point in the application's execution where an aspect can be applied to inject additional behavior, such as method invocations or object instantiations.
Advice: An advice is an action taken by an aspect at a specific join point. It includes methods executed when a matching join point is reached in the application.
Pointcut: Pointcuts define the criteria for selecting join points where advice should be applied, allowing for precise control over aspect behavior.
Target Object: The target object represents the original component being advised by one or more aspects, encapsulating the primary functionality provided by the application.
AOP Proxy: Proxies act as intermediaries between the client and the target object, intercepting method invocations and applying the appropriate advice defined in associated aspects.
Weaving: Weaving is the process of applying aspects to join points in the codebase. Spring AOP performs weaving at runtime, seamlessly integrating aspects into the target object's behavior.
AOP Advice Types:
Spring AOP offers various advice types to intervene at different stages of method execution:
Before advice: Executed before a join point, allowing for pre-processing tasks.
After returning advice: Executed after a join point completes successfully, ideal for post-processing tasks.
After throwing advice: Executed if a join point throws an exception, enabling error handling.
After advice: Executed regardless of the outcome of the join point, suitable for cleanup tasks.
Around advice: Wraps around the target method, allowing for custom pre-processing, post-processing, or modification of method behavior.
Supported Pointcut Designators:
Spring AOP supports AspectJ pointcut designators for precise method selection:
execution(): Matches method execution join points.
within(): Matches method executions within specified packages.
this(): Matches method executions on beans whose type matches the current AOP proxy.
target(): Matches method executions on beans that match the current AOP proxy.
args(): Matches method executions where the arguments of the method match specified types.
@target(): Matches method executions on beans that match specified types of AOP proxy.
@args(): Matches method executions where one of the arguments of the method matches specified types of AOP proxy.
@within(): Matches method executions on beans within specified annotated types.
@annotation(): Matches method executions annotated with specified annotations.
Implementing AOP in a Spring Boot Application:
Follow these steps to seamlessly integrate AOP into your Spring Boot project:
Add the Spring Boot starter AOP dependency to the project: Open your pom.xml file and add the following dependency within the <dependencies> section:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-aop</artifactId>
</dependency>
Create an aspect class annotated with @Aspect: Create a new Java class in your project's source directory and annotate it with @Aspect.
Define pointcut expressions using @Pointcut: Within your aspect class, create methods annotated with @Pointcut to define pointcut expressions.
Implement advice methods using annotations like @Before, @After, or @Around: Define methods within the aspect class annotated with advice annotations to specify the type of advice.
The aspect is automatically detected and applied to the appropriate join points in the application by Spring Boot.
Example:
Illustrate the implementation of AOP with a practical example:
// Define services
public class AdminService {
public void addAdmin(String adminName) {
System.out.println("Adding admin: " + adminName);
}
// Other methods of AdminService
} @Service
public class UserService {
public void addUser(String username) {
System.out.println("Adding user: " + username);
}
// Other methods of UserService
}
// Create an aspect class
@Aspect
@Component
public class LoggingAspect {
@Pointcut("execution(* com.example.myapp.service.UserService.*(..))")
public void userServiceMethods() {}
@Pointcut("execution(* com.example.myapp.service.AdminService.*(..))")
public void adminServiceMethods() {}
@Before("userServiceMethods() || adminServiceMethods()")
public void logServiceMethodCall() {
System.out.println("Method in UserService or AdminService is being called...");
}
}
In this example,
We defined two methods annotated with @Pointcut: userServiceMethods() to select methods of the UserService service and adminServiceMethods() to select methods of the AdminService service. We then use @Before to define advice that will be executed before the execution of all methods of the UserService or AdminService service.
Now, when you call any method of UserService or AdminService, the LoggingAspect aspect will be automatically applied due to the @Pointcut configuration, and the logServiceMethodCall() method will be executed before the execution of each called method. This allows you to monitor calls to multiple services without duplicating advice code.
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.context.ConfigurableApplicationContext ;
@SpringBootApplication
@EnableAspectJAutoProxy(proxyTargetClass = true)
public class MainApplication {
public static void main(String[] args) {
ConfigurableApplicationContext context = SpringApplication.run(MainApplication.class, args);
// Retrieve the UserService bean from the context
UserService userService = context.getBean(UserService.class);
// Call the addUser() method of the UserService.
userService.addUser(“John”);
// Retrieve the AdminService bean from the context
AdminService adminService = context.getBean(AdminService.class);
// Call the addAdmin() method of the AdminService
adminService.addAdmin(“Admin”);
// Close the Spring application context
context.close();
}
}
After executing this application, you should obtain the following output:
Method in UserService or AdminService is being called…
Adding user: John
Method in UserService or AdminService is being called…
Adding admin: Admin
Conclusion:
In conclusion, leveraging Spring AOP enhances code quality and organization in Java applications. By encapsulating cross-cutting concerns and providing a systematic approach to aspect-oriented programming, Spring AOP simplifies development and maintenance tasks, ultimately improving the overall quality of Java applications.